
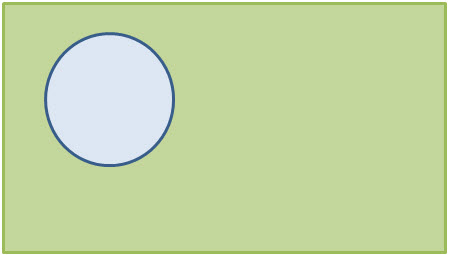
In the image above you can see we have drawn 3 dashed lines. So how do we check if a point is inside a polygon? We simply draw a line from the point to somewhere external to the polygon. So if we check to see if a corner point is inside the other polygon we know they are colliding There are some edge cases where the points do not fall within the other polygon however this is not covered in this guide as it is only an introduction to collision detection. Let’s take a look at some polygons that are colliding and see what we can tell about polygon collisions.įrom this image you can see that if a convex polygon is colliding then at least 1 of the corners(vertices) is inside the other polygon. Polygons don’t have a set radius or a set width and height so we can’t use the previous methods.

So we covered circles and rectangles but how about polygons. So first we need to get the position of all sides: In our example we will be using the position as the bottom left corner but you could use the center of the rectangle with a little extra math.
CIRCLE RECTANGLE SHAPE HOW TO
so now we know how to check if a rectangle is in a rectangle but how do we do this in code?įirst, we must find out where the sides of the rectangle are and we do that using the width, height and position of the rectangle. well, it means nothing they still haven’t collided only one side of each is inside and for a collision 2 sides must be inside the other rectangle.Ī collision has happened and both sides of each rectangle are inside the other rectangle. Now you can see that one side of each rectangle is inside the other and this means. This says the rectangle is not colliding. You can see by the dotted lines that neither the bottom nor right edge fall inside the other rectangle. Here you can see 2 rectangles that have not collided yet. Lets take a look at two rectangles about to collide: So how do we check if 2 rectangles are colliding? we check to see if 2 of the sides fall in between the other rectangle. Rectangles are often used to define a collision boxes for entities that don’t require precise detection.
CIRCLE RECTANGLE SHAPE CODE
The code for this is:Ī rectangle in computing usually refers to a shape with 4 corners and 2 parallel sides and is a common shape for use in collision detection due to its simplicity to make and detect collisions with. Now we do the same on the y axis using circle’s y position – circle2’s y position. For us to get the x axis length values we would take the absolute value(non negative) of circle 1’s x position – circle 2’s x position this gives us one side of the triangle. The Pythagorean theorem states that the hypotenuse (the side opposite the right angle) is always the square root of side 1 squared + side 2 squared. Now we have a right angled triangle we can use Pythagorean theorem to work out the distance. and not just any triangle, a right angled triangle. So how do we work out the distance between the 2 circles? If we draw a line on the x axis from 1 circle to the other and one on the y axis we get this: So now we know if the distance between the circles is smaller than the combined radius of both circles then the circles are colliding. Any further apart and they would not be touching and any closer and they would be inside each other. If you look at this image you can see the radius of circle 1 (the red line) and the radius of circle2 (the blue line) when both circle touch create a line between the centers of both circles. So how do we detect of 2 circles are colliding? Well, lets take a look at 2 circles colliding and see what we can tell:


But if you don’t really need all the physics processing that comes with Box2D then you’re adding a lot of bloat to your game when it could be done with some nice algorithms shown in this guide. well yes, you could and it would work pretty darn good. Surely you could use a framework like Box2D to do all the collision detection for you. This calls for collision detection algorithms. So you’re making a game and you want to check if your character has bonked an enemy. Collision Detection – Circles, Rectangles and Polygons
